MigaPushAPI
Orientation
This module is very simple, and allows external applications to:
- send individual push notifications via customer email
- fetch user list
Settings
You can access the Settings tab from app builder frontend. From Vertical menu on the left, click on Module section and so “Miga Push Api”.
You have to set only the maximum per minute sends a push to the customer. Also, user can regenerate token if any need.
Note:
- API URL For Send Individual Push use with post request and also five fields are required {`app_id`, `token`, `message`, `subject`, ’email’} but “email” is the most important field
- API URL Get Users List use with post request and also two fields are required {`app_id`, `token`}
User List
Description
Fetch all the users available for sending Push notifications.
$endpoint = "https://www.domain.com/migapushapi/public_apiusers/init"
Request
Param | Type |
---|---|
app_id | int |
token | string |
Response
{
“error”: null,
“data”: [
{
“email”: “user.com”,
“firstname”: “user”,
“lastname”: “x”
},
{
“email”: “user2.com”,
“firstname”: “user2”,
“lastname”: “x”
}
]
}
Example
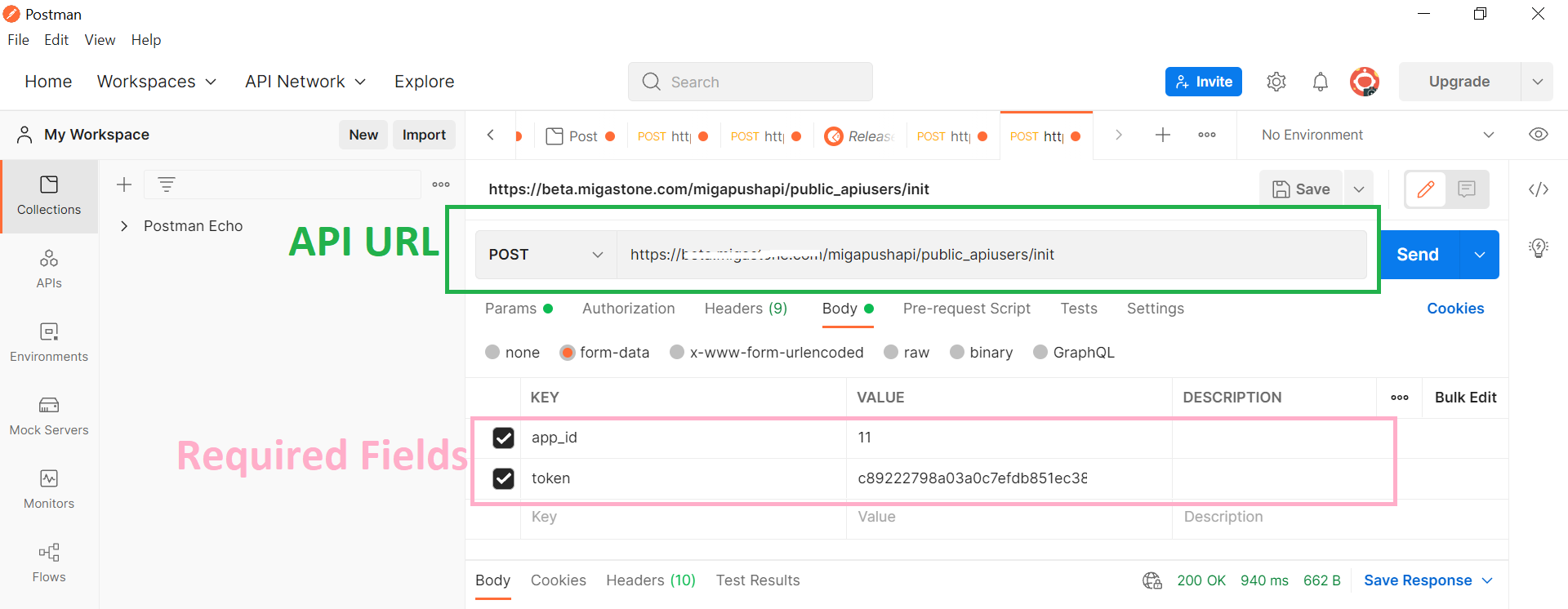
Send Individual Push
Description
Sends an individual push notification via user email.
$endpoint = "http://www.domain.com/migapushapi/public_api/init"
Request
Param | Type | Details |
---|---|---|
subject * | string | Push title |
message * | string | Push message |
app_id * | int | |
token * | string | |
email * | string | |
devices | string | all , android , ios |
open_url | int | If set to 1 url will be opened in app |
url | string | Along with open_url set to 1, the url to open |
cover | string | base64 encoded image to display as a cover, must be png or jpg. For Example: data:image/jpeg;base64,/9j/4AAQSkZJRgABAQEASABIAAD……… |
Cover Image BASE64 Conversion Steps.
- Go to BASE64 Conversion link
- Choose a cover image (jpg, png)
- Select output type format (Data URI — data:content/type;base64)
- Click on encode image button.
Example of PHP implementation
For Get User List
<?php
$url = ‘https://domain.com/migapushapi/public_apiusers/init’;
$data = array(
“app_id” => “11”,
“token” => “c89222798a03a0c7efdb851ec38…….”,
);
$curlHandler = curl_init();
curl_setopt_array($curlHandler, [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
/**
* Specify POST method
*/
CURLOPT_POST => true,
/**
* Specify request content
*/
CURLOPT_POSTFIELDS => $data,
]);
$response = curl_exec($curlHandler);
curl_close($curlHandler);
echo($response);
?>
For Send Push Notification
<?php
$url = “https://domain.com/migapushapi/public_api/init”;
$data = array(
“app_id” => “11”,
“token” => “c892227…….”,
“message” => “Message……”,
“subject” => “Subject……..”,
“email” => “email@gmail.com”,
“devices” => “android”,
“open_url”=>1,
“url” => “https://domain.com/api/push/”,
“cover”=>”data:image/png;base64,iVBORw0KGgoAAAANSUhE……………..”
);
$curlHandler = curl_init();
curl_setopt_array($curlHandler, [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
/**
* Specify POST method
*/
CURLOPT_POST => true,
/**
* Specify request content
*/
CURLOPT_POSTFIELDS => $data,
]);
$response = curl_exec($curlHandler);
curl_close($curlHandler);
echo($response);
?>
Example of Javascript implementation
For Get User List
<script>
$.ajax({
url: “https://domain.com/migapushapi/public_apiusers/init”,
type: “post”,
data: {
“app_id”: “11”,
“token”: “c89222798a03a……”
} ,
success: function (response) {
console.log(response);
},
error: function(jqXHR, textStatus, errorThrown) {
console.log(textStatus, errorThrown);
}
});
</script>
For Send Push Notification
<script>
$.ajax({
url: “https://domain.com/migapushapi/public_apiusers/init”,
type: “post”,
data: {
“app_id” : “11”,
“token” : “c892227…….”,
“message” : “Message……”,
“subject” : “Subject……..”,
“email” : “email@gmail.com”,
“devices” : “android”,
“open_url” :1,
“url” : “https://domain.com/api/push/”,
“cover”:”data:image/png;base64,iVBORw0KGgoAAAANSUhE……………..”
},
success: function (response) {
console.log(response);
},
error: function(jqXHR, textStatus, errorThrown) {
console.log(textStatus, errorThrown);
}
});
</script>
Success Response
{
“success”: true,
“response”: 1
}
All Response
Response | Details |
---|---|
1 | Successful |
0 | Wrong/Missing Token |
2 | App ID is missing |
3 | Email is missing” |
4 | Email User hasn’t a push token |
5 | Message field is empty |
6 | Subject is missing |
7 | Url is missing (if field OpenUrl is 1 value and Url is empty) |
8 | Wrong Cover Image base64 encoded image to display as a cover, must be png or jpg |
9 | Invalid Request |
10 | Invalid Parameter(s) Found. |
11 | Already user send max push plz wait one minute |
12 | Email does not exists |
13 | Cover image size must be smaller than 1MB |
14 | Cover image must be jpg, png |
Example